ALL LESSONS![]() |
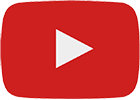
Lesson 01 - Display Objects
Theory
People often learn to code by seeing results in a text console (yawn). With ZIM, we work on the HTML Canvas and it is very visual (yay!). We will practice coding with visual elements called Display Objects! The Ornamator is an example of the type of apps we can make. Here are more Examples.
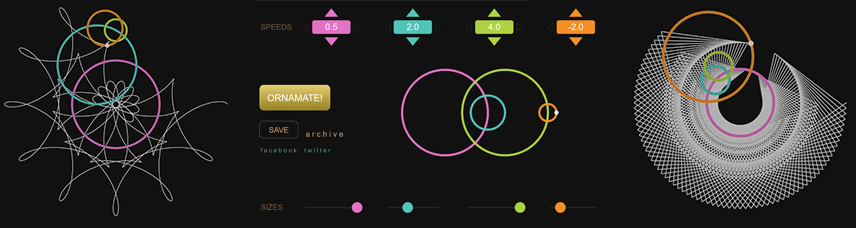
Display Objects are things that we can see in our app such as shapes, components, bitmaps (images) and sprites as well as containers to hold these. Display Objects must be added to the stage to be seen. The stage is the base Display Object that the user sees.
In traditional HTML, Display Objects are made from <tags> and are organized in a hierarchy (tree) called the Document Object Model (DOM) and viewed in the <body>. On the canvas, Display Objects are made from classes listed in the Display Objects module of the ZIM Docs and organized in a hierarchy called the Bitmap Object Model (BOM) on the stage. ZIM is built on CreateJS which gives us the BOM and all of this is built with JavaScript.
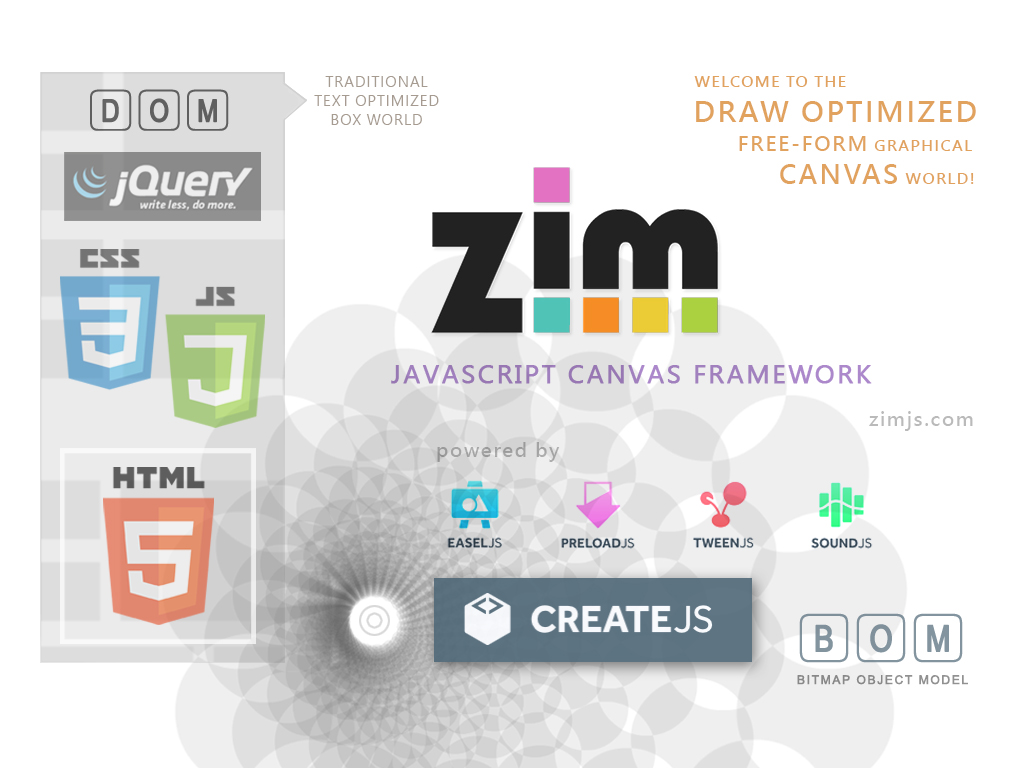
Let's go to a REFERENCE section where we define the parts of coding that we will be using.
Reference
The ZIM Skool Reference sections have explanations of the code we will be using with important words highlighted in orange. There are links to information videos - that can also be found in the ZIM Learn page. Some of these videos are older when we used the zim namespace or before we use the chainable methods. See the Tips page for the current way we code.
Classes
► What IZ Object -
What IZ Class -
What IZ Property -
What IZ Method
Classes are the template from which an object (noun) is made. They define the properties and methods of the object.
Properties describe the object or is something that the object has (adjectives).
Methods are what the object does or has done to it (verbs).
All of this is called Object Oriented Programming (OOP) and it is the metaphor we use for coding.
Objects ► What IZ Object
To make a new object from a class we use the new keyword followed by the class name (which by convention starts with a capital letter).
We can make many objects from the same class and each object has its own properties and we can run methods on individual objects.
new Button(100, 50, "CLICK");
Parameters
When we make an object we use parameters to tell the class extra information
such as what size, color, value, etc. the object will have.
These are passed in as arguments in the round brackets () of the class and separated by commas (,) in the order specified in the Docs.
In the Button example above, 100 and 50 are arguments passed in to the width and height parameter of the button. Often we just call them parameters. "CLICK" is collected in the label parameter.
Methods also have round brackets and parameters but properties do not. As we will see in a later lesson, plain functions have parameters as well.
Variables ► What IZ Variable
We can store an object in a variable which is like label or a reference to the object so we can access the object later.
We use the var "keyword" to declare the variable followed by an "identifier" (name of the variable), then the assignment "operator" (=) followed by the object "expression".
var circle = new Circle(20, "red");
Note: in JavaScript 6 (ES6) we have the let and const keywords. Going forward, the let keyword will be used instead of var as it has more specific scoping. The const keyword is used when the object being stored will not be replaced with another object. These differences can be considered later in your programming career!
// in ES6 a const stores an object that does not get replaced by another object const num = 10; num = 5; // error! Cannot reassign a constant // below is okay - we did not change the object, we just "mutated" it - cool! const circle = new Circle(10, "red"); circle.color = "blue";
scope ► What IZ Scope
A variable var is available within the { } of the function it is declared or if not in a function, then it is available globally.
The availablility is called scope and we can only ask for the variable's value or assign a new value within its scope.
We see functions in Lesson 03 and will give examples of scope there.
Note: as mentioned, in JavaScript 6 (ES6) we have the const and let declarations. These are scoped differently. They are available within whatever { } they are declared. This could be a conditional if ( ) { } or a loop for ( ) { }. We see these in future lessons too. Let's leave the code examples until later as we are just introducing the concept of scope here.
Statements
► What IZ Syntax -
What IZ Operator -
What IZ Expression -
What IZ Statement
A statement is like a step in the instructions.
Statements are made up of parts such as keywords, identifiers, operators and expressions and end in a semi-colon (;) terminator.
There is a specific syntax that must be followed otherwise we get an error.
Statements run in order unless functions, conditionals or loops are involved (more on those later).
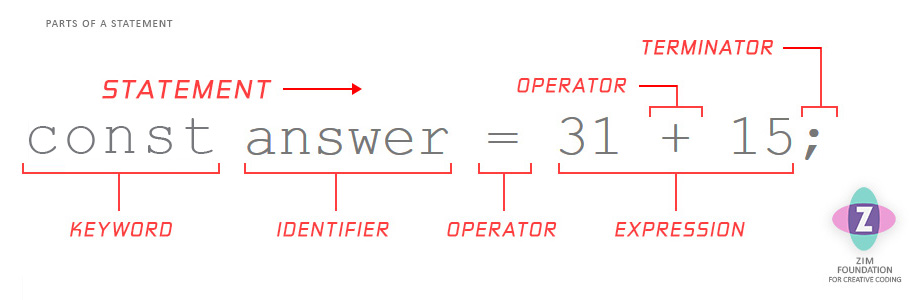
Usually a statement is on one line but can be on multiple lines or there can be any number of statements on one line. Traditionally, for example, we would store an object in a variable in one statement. Then on the next line, in the next statement, we would use the variable name to set a property or call a method, etc.
Dot Syntax ► What IZ Dot
We use a dot (.) between the object and any properties or methods that we want to use.
circle.addTo(stage); // addTo() is a method circle.x = 200; // set the x and y properties for position circle.y = 100; // the two slashes mean a comment
Chaining ► Bubbling Chaining
Chaining allows us to call one or more methods of an object one after the other separated by a dot (.) all in ONE statement.
This makes less code so we currently use chaining in ZIM code.
Often, we do not need to store the object in a variable - we can just use chaining.
new Circle(20, "red").loc(200,100);
Properties such as x and y, alpha, scale (scaleX, scaleY), rotation, etc. cannot be chained. So ZIM has provided short replacement methods that can be chained. These include loc(), pos(), mov(), alp(), rot(), sca(), etc.
ZIM also provides addTo(), center(), centerReg() and removeFrom() chainable methods to add objects to the stage or containers or remove them. Other handy chainable methods are drag() and animate() and more can be found in the Docs under the Methods module.
We can place chained methods on multiple lines and indent to make the code more readable. When we do, remember to put the semi-colon only at the end of the statement.
new Blob() .center() .sca(.5) // half scale .drag();
Containers ► Container Basics - Container Basics Part 2
A Container is a DisplayObject that lets you group DisplayObjects together (including other containers).
The container itself is invisible.
You can start a container with no dimensions in which case the container will grow in size as contents are added.
Or you can start a container with dimensions in which case it will keep these dimensions unless setBounds(null) is called.
You can move, rotate, scale, set alpha on a container and all its contents will affected as one. There are other advantages too that we explore in future lessons. Each container has its own x and y coordinate system inside.
// MAKE A CONTAINER // here we do not start the container with dimension const holder = new Container().loc(200,100); // add a Rectangle to the container // the rectangle will be added at 0,0 inside the container // so will appear at position 200,100 on the stage new Rectangle(100,100,red).addTo(holder); // locate the second rectangle inside the container // the second rectangle will appear at 400,300 on the stage new Rectangle(100,100,blue).loc(200,200,holder); holder.drag(); // will let the individual contents be dragged separately // or holder.drag({all:true}); // will drag the whole container (both rectangles together) holder.alp(.5); // will set the alpha of the whole container to half holder.sca(.5); // will scale the whole container including the rectangles to half size zog(holder.numChildren); // will log 2 zog(holder.width); // 150 (rect of 100 at position 200 inside container = 300 then scaled in half) holder.getChildAt(0); // will get a reference to the first red (or just assign it to a variable to start) holder.removeFrom(); // will remove the container from the stage (including the rectangles) // MAKE A DIMENSIONED CONTAINER // makes a container the same size as the stage and add it to the stage const scene = new Container(stageW, stageH).addTo(); // center a Circle on the scene // the circle will appear in the center of the stage // but if we want to we could remove this scene and add another // this is how we handle multiple pages // or use the Page() component that is just a Container with a background color new Circle().center(scene);
Let's go to a PRACTICE section where we will see all these terms in action and make Display Objects from Classes.
Practice
The ZIM Skool Practice sections have editable text areas for you to practice coding.
Instructions
Click the practice bars below to expand the exercises.
Inside, there are blue numbered boxes that provide different code to try.
Type the code in the second text box - make sure to use the same syntax.
Then press the TEST button or use ctrl S (PC) or command S (mac) to see the results in the ZIM practice window.
Summary
At ZIM Skool, we code with a visual result in JavaScript for the Canvas. We start with Display Objects such as shapes and components that are made from classes and are placed on the stage. We use properties and methods to transform the Display Objects.
In the next lesson, we look at Configuration Objects to help us with our many parameters. We also explore animation!
LESSON 02 - CONFIG OBJECTS AND ANIMATION
VIDEOS
ALL LESSONS![]() |