BADGE 6
BACKMake a dangling chain and action button!
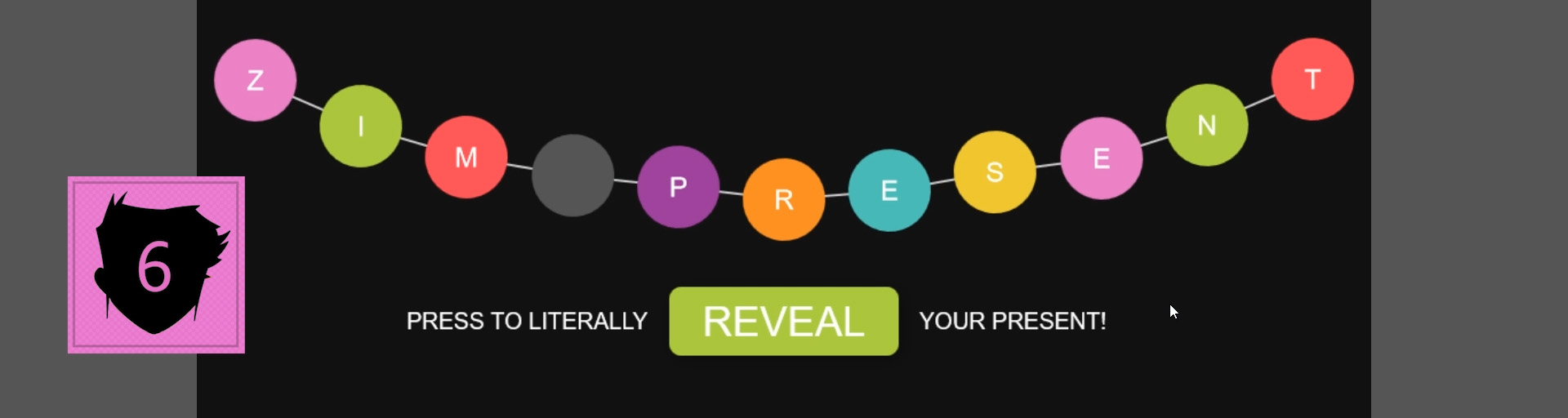
For our last Badge, we will stop the physics and animate all the balls up to a dangly chain.
We will then animate in a message and button to link to the Badges tutorial for the app.
55 a) INSIDE and at the END of the done() function, use the dispose() method of physics to stop any physics calculations.
The ZIM shapes will all stay where they are.
55 b) Use the animate() method of the wrapping to fade the alpha out - with parameters of {alpha:0},700.
55 c) Use the animate() method of the walls to fade the alpha out - with parameters of {alpha:0},700.
HIGHLIGHT MORE ANSWER
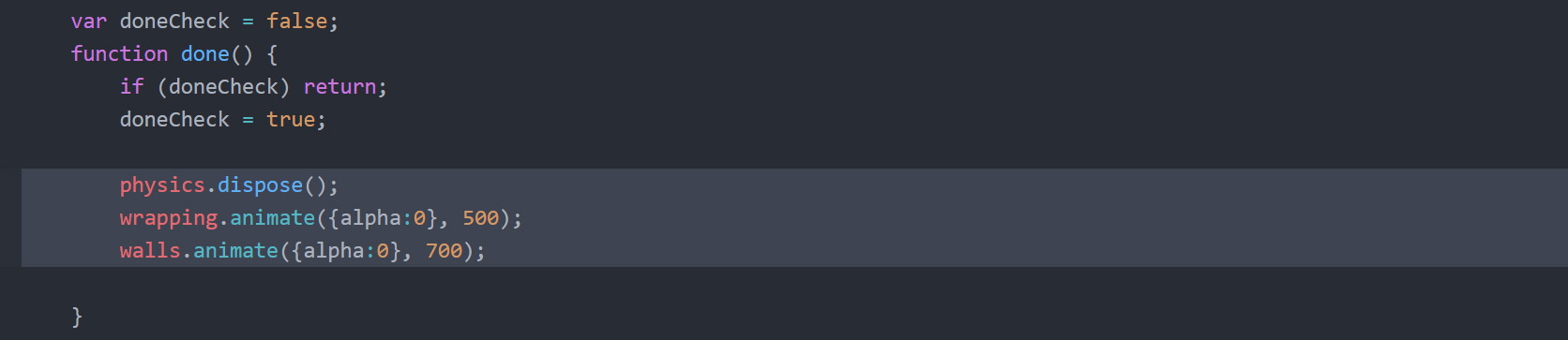
Next, we will animate() the balls up towards the top with an animation sequence.
This animates each ball in the sequence the same but delayed in time.
If we animate to {y:100} all the balls would go to the same height and the chain would be straight.
We want the chain to droop a little like in real life.
So we will use a ZIM VEE value as the y value of the animation.
ZIM VEE uses zik() to pick a value for each animation sequence.
Click the link for zik() to see the various options for ZIM VEE values.
An example would be passing {y:{min:100,max:200}}
to animate each ball to a random number between 100 and 200.
Or {y:[100,150,200]} for a random choice of these values.
We want to pass a function with a little equation that has values which make a parabola.
A function that returns a value is another option for a ZIM VEE value.
We will set a count value outside the function to help our little equation.
56 a) INSIDE the done() function make a variable called count and assign -6.
Each time we run the sequence function we will increase this and it will go from -6 to positive 5 (as we have 11 balls).
56 b) Make a variable called colors and assign an Array of the following colors: frame.pink, frame.green, frame.red, frame.grey, frame.purple, frame.orange, frame.blue, frame.yellow.
56 c) Use the animate() method of balls with a parameter of a configuration object with the following properties:
- obj:{}
- INSIDE the object we will have the following two properties to animate:
- y:function(){}
- INSIDE the function is our little equation:
- count++; to increase our count
- return -count*count*4; to adjust the y value each time
- scale:1.2 to increase the size of the balls a little
- time:900
- ease:"backOut"
- sequence:100 - the time between animating each ball
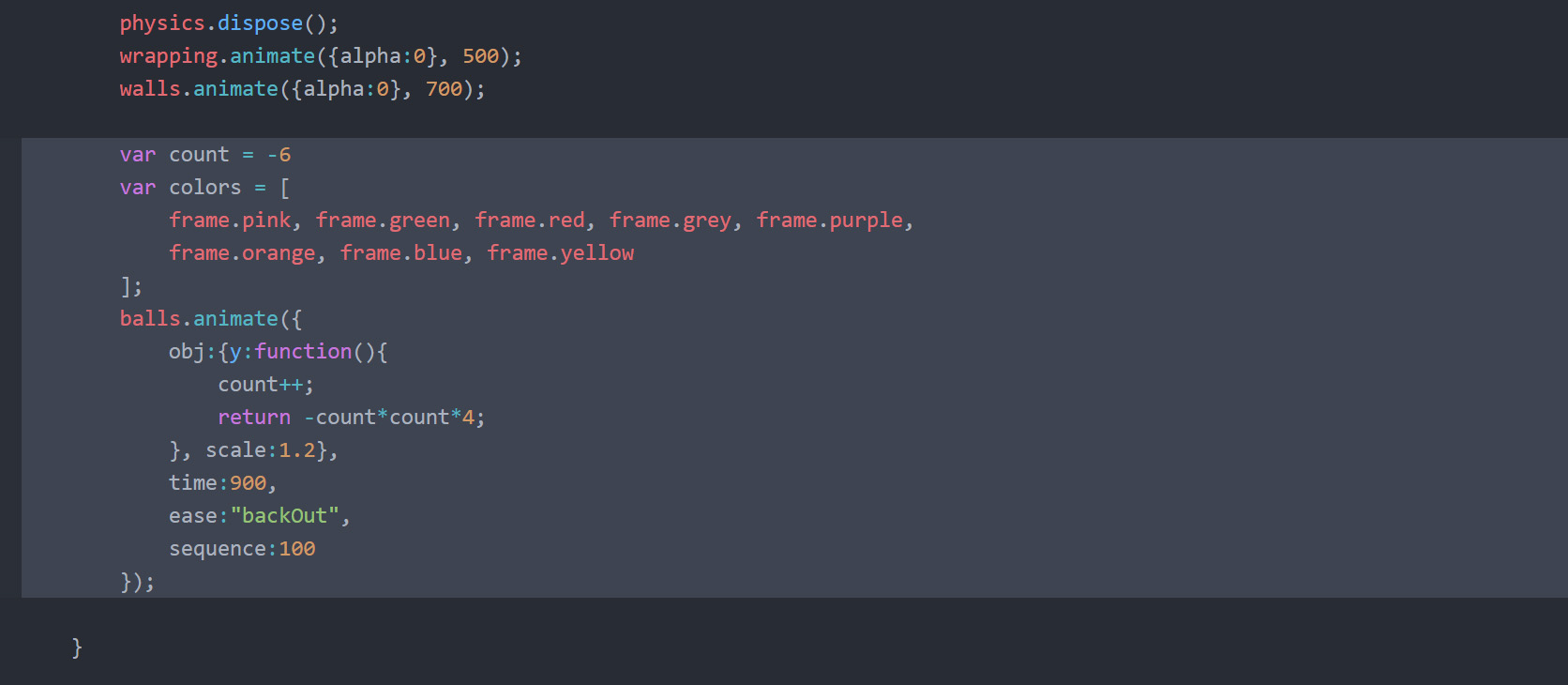
ZIM Learn References
57 a) ADD another property to the animation configuration object that is a call
property with a value of a function(){}.
57 b) INSIDE the ( ) collect a parameter we will call target. This is the object that is currently ending its animation. INSIDE the function use the wiggle() method of the target. Put the object first (target) followed by the dot (.) and the method (wiggle()) passing the following parameters (not a configuration object - just plain parameter values):
57 c) Set the color property of the target to a color from the colors array. Let's use the colors in sequence rather than a random color. Put the object (target) followed by the dot (.) then assign (=) the array (colors) and then the array access operator [ ]. Inside the [ ] put balls.getChildIndex(target)%colors.length.
57 b) INSIDE the ( ) collect a parameter we will call target. This is the object that is currently ending its animation. INSIDE the function use the wiggle() method of the target. Put the object first (target) followed by the dot (.) and the method (wiggle()) passing the following parameters (not a configuration object - just plain parameter values):
- "y" - the property we will wiggle
- target.y - the base value of the property
- 2 - the minimum we will change the property
- 5 - the maximum we will change the property
- 200 - the minimum time to change the property
- "y" - the maximum time to change the property
57 c) Set the color property of the target to a color from the colors array. Let's use the colors in sequence rather than a random color. Put the object (target) followed by the dot (.) then assign (=) the array (colors) and then the array access operator [ ]. Inside the [ ] put balls.getChildIndex(target)%colors.length.
We want each ball to get a color and then when all the colors are used up, start over again.
We will use the index of the ball within its container. The index will go from 0-10 for 11 balls.
We only have 8 colors. A common technique to use in this case is the modulus which has the format of:
count%divisor
This will evaluate to the remainder of count/divisor with the count being used when count is less than the divisor. So the result of ball index % number of colors will be: 0,1,2,3,4,5,6,7,0,1,2
HIGHLIGHT MORE ANSWER
count%divisor
This will evaluate to the remainder of count/divisor with the count being used when count is less than the divisor. So the result of ball index % number of colors will be: 0,1,2,3,4,5,6,7,0,1,2
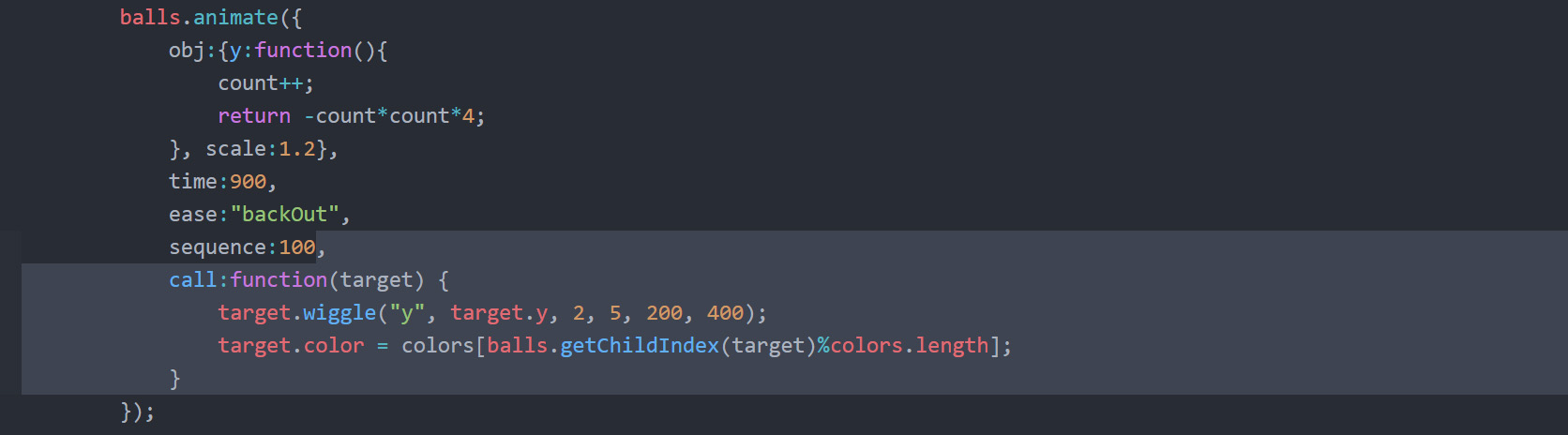
ZIM Learn References
58 a) Make a new Rectangle() with parameters of
stageW,stageH,frame.darker and chain on
addTo() with parameters of stage,0
to put the shape behind everything.
Chain on alp() with a value of 0.
Chain on animate() with a configuration object as follows:
HIGHLIGHT MORE ANSWER
- obj:{alpha:1}
- wait:2500
- waitedCall:makeButton
- time:1000
HIGHLIGHT MORE ANSWER
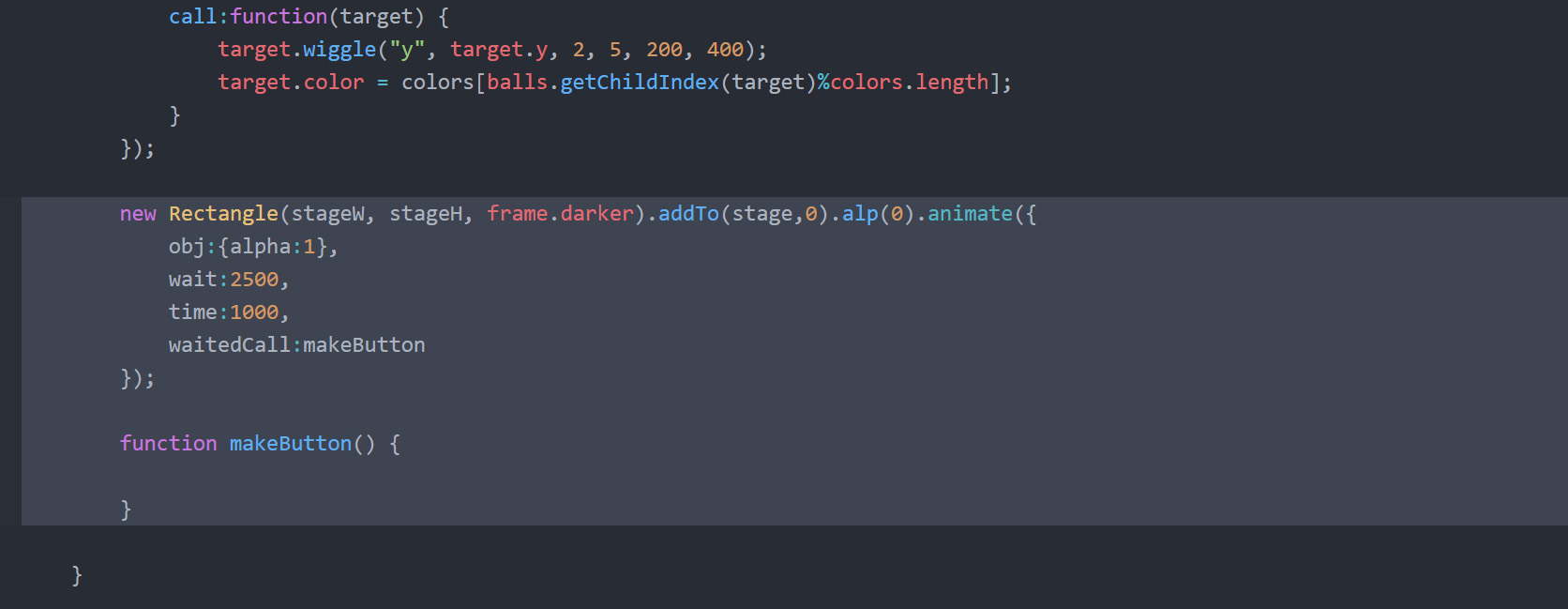
59 a) In the makeButton function { }, make a variable called final and assign a new Container() with parameters stageW,stageH.
Chain on a centerReg() with parameter stage.
59 b) Make a new Label() with a parameter of a configuration object with the following properties:
59 c) Make a new Button() with a parameter of a configuration object with the following properties:
59 d) Make a new Label() with a parameter of a configuration object with the following properties:
HIGHLIGHT MORE ANSWER
59 b) Make a new Label() with a parameter of a configuration object with the following properties:
- text:"PRESS TO LITERALLY"
- color:frame.white
- size:20
59 c) Make a new Button() with a parameter of a configuration object with the following properties:
- label:"REVEAL"
- corner:10
- color:frame.green
- rollColor:frame.red
59 d) Make a new Label() with a parameter of a configuration object with the following properties:
- text:"YOUR PRESENT!"
- color:frame.white
- size:20
HIGHLIGHT MORE ANSWER
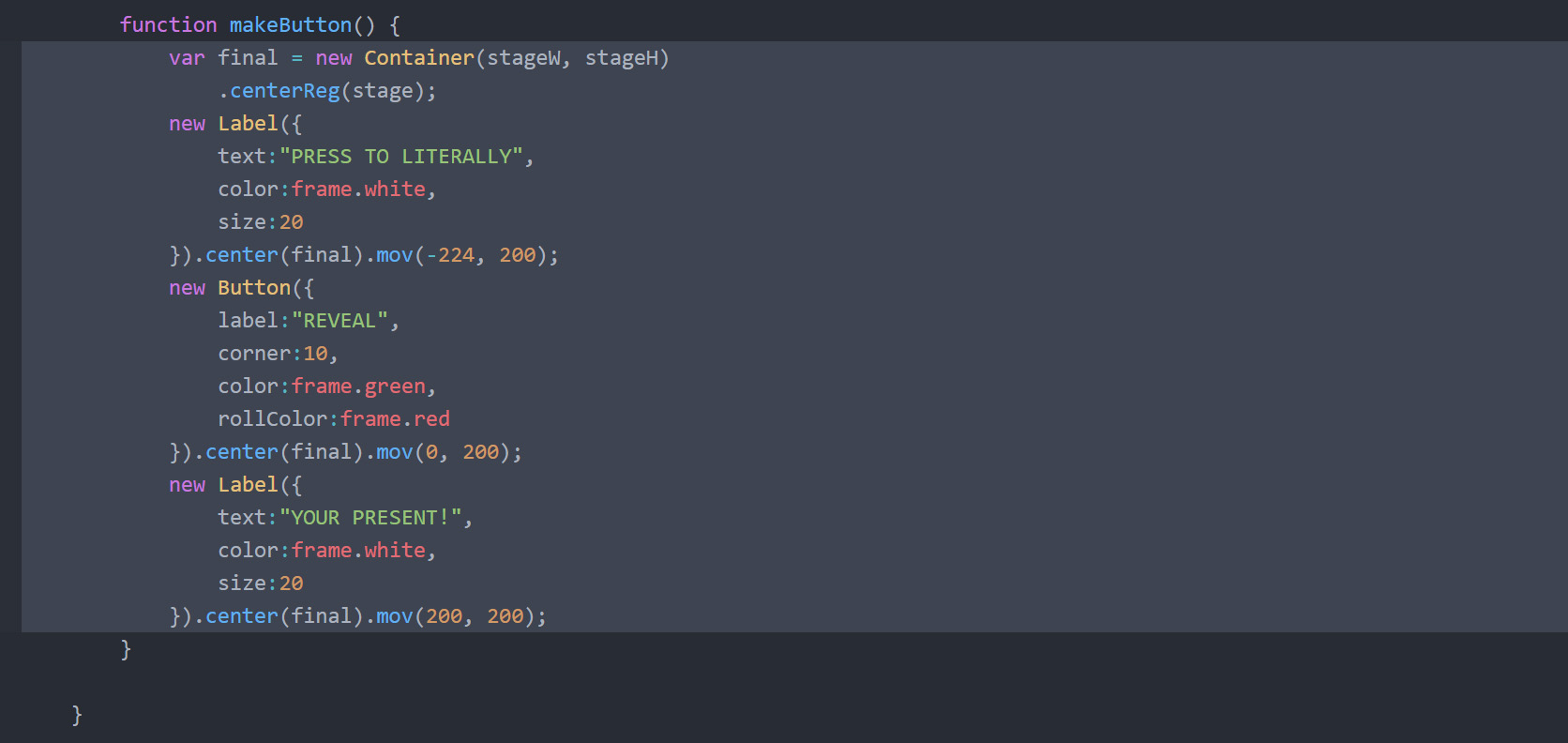
ZIM Learn References
60. Chain on to the Button the on() event with parameters "click",function(){}.
Remember to remove the semi-colon (;) from the end of the Button statement.
INSIDE the function { } use the zgo() method
with a parameter of "https://zimjs.com/badges/phys.html".
This will take the user to the Badges page when they click on the button.
It is risky to chain an on() method as it does not return the object.
This means that if the statement were assigned to a variable it would not contain
a reference to the Button we made but rather the function identifier that the on() method returns.
Here, we will not need the button again - but if we changed code so that we did, we would
have to remember to unchain the on() method.
A good rule of thumb is to not chain the on() method.
To accommodate that here, we would have to assign the Button to a variable and then put the on() method on the variable.
HIGHLIGHT MORE ANSWER
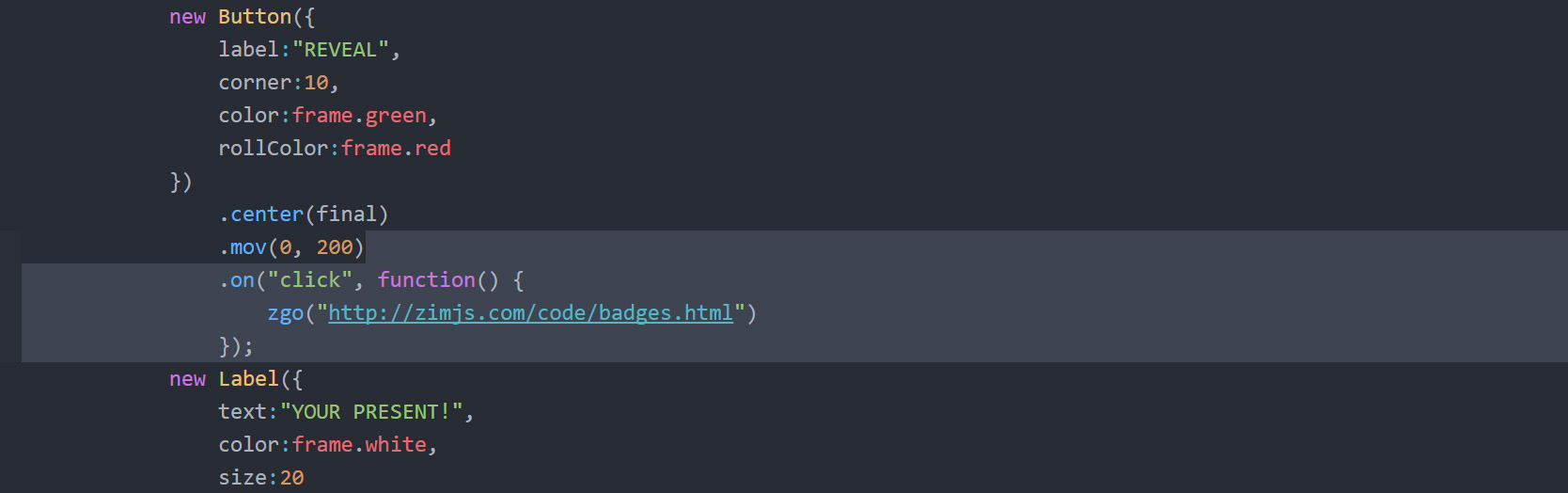
ZIM Learn References
61. We are going to animate in the scaleX of the final container.
Put the object (final) followed by the dot (.) then sca() with parameters 0,1
to give the container no scale in the x but a 1 scale in the y.
Chain on animate() with a parameter of a configuration object with the following properties:
- obj:{scaleX:1} - to animate in the scaleX
- time:500
- wait:500
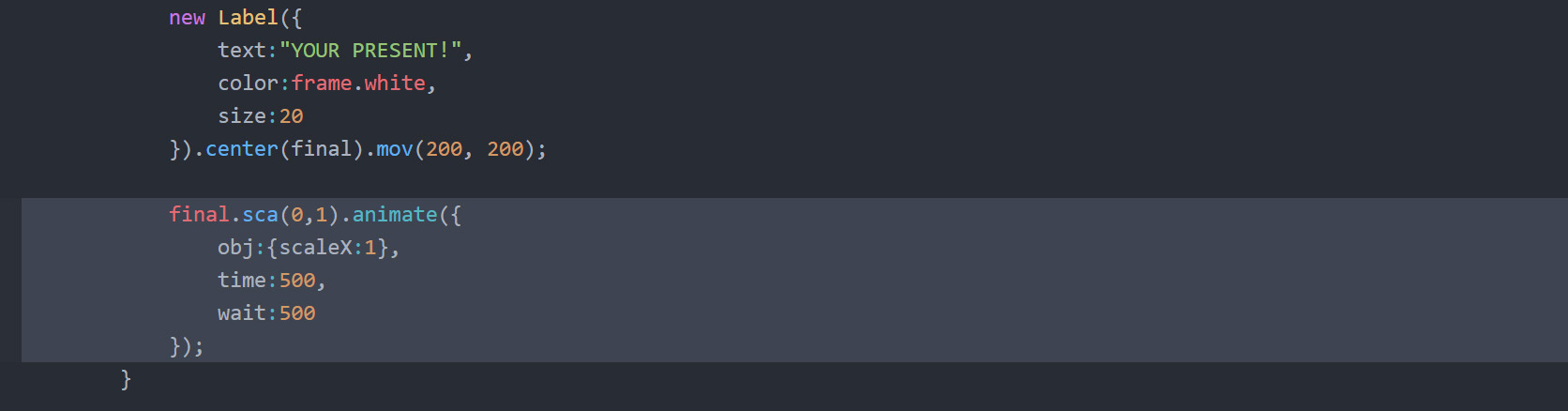
62. The last step is to go UP to where we made our new Physics(frame)
and set the third parameter to 5.
This reduces the gravity to tune our application and make it a bit more gentle.
Pass null in as the second parameter as we will just use the default borders.
HIGHLIGHT ANSWER
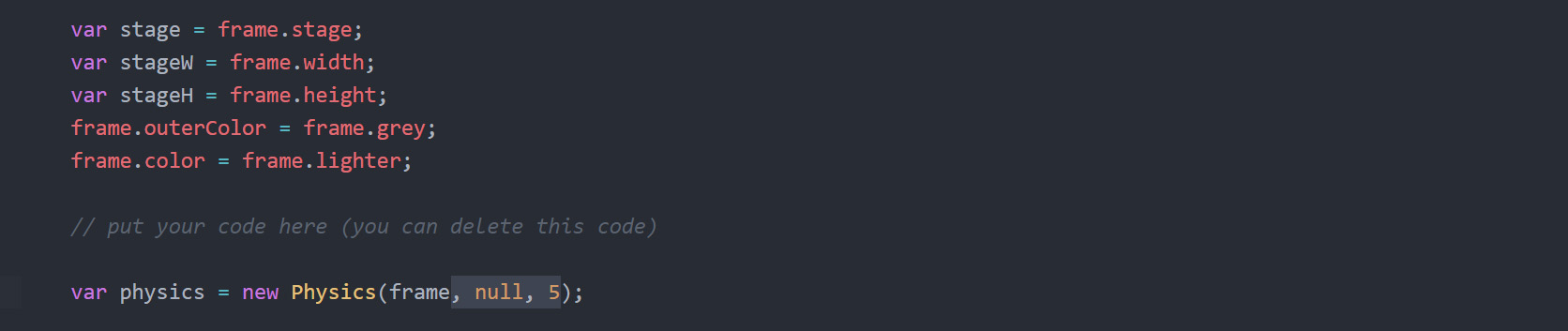
CONCLUSION: Physics can add magical movement to your Interactive Media.
It can also make many things easier like mazes, side scrollers, etc.
The ZIM Physics module makes physics very easy to use with ZIM.
Here is an example of making a physics rectangle without it:
This is a very real tutorial with much more than just physics. It demonstrates many of the usual Interactive Media techniques found throughout the MORE links. These include:
Dr. Abstract and Pragma
var b2Vec2 = Box2D.Common.Math.b2Vec2; var b2BodyDef = Box2D.Dynamics.b2BodyDef; var b2Body = Box2D.Dynamics.b2Body; var b2FixtureDef = Box2D.Dynamics.b2FixtureDef; var b2Fixture = Box2D.Dynamics.b2Fixture; var b2World = Box2D.Dynamics.b2World; var b2PolygonShape = Box2D.Collision.Shapes.b2PolygonShape; var SCALE = 30; var boxW = 200 / SCALE; var boxH = 200 / SCALE; // define the box var boxBodyDef = new b2BodyDef(); // for some reason classes are lowercase boxBodyDef.type = b2Body.b2_dynamicBody; boxBodyDef.position.Set(300/SCALE, 400/SCALE); // for some reasons methods are uppercase // create a body for the box boxBody = world.CreateBody(boxBodyDef); // create a shape for the box var boxBox = new b2PolygonShape(); boxBox.SetAsBox(boxW/2, boxH/2); // create a fixture to make the box body take the shape var boxFixtureDef = new b2FixtureDef(); boxFixtureDef.shape = boxBox; boxFixtureDef.density = 1; boxFixtureDef.friction = 1; boxBody.CreateFixture(boxFixtureDef);So, you can see that we have saved you some work considering that this is ONE LINE in ZIM Physics! The mapping from physics to ZIM has been made easy and the dragging and the running of the world, etc.
This is a very real tutorial with much more than just physics. It demonstrates many of the usual Interactive Media techniques found throughout the MORE links. These include:
- Animation with waits and calls
- Configuration objects or parameters
- Looping and hitTests
- Tickers and timeouts
- Containers, arrays and modulus
- Variables, conditionals and function
- Classes, objects, properties and methods
- Events and event objects
- Buttons and Labels
- Particle emitters and wiggling
- Dynamic drawing in shapes
- Principles of abstraction
Dr. Abstract and Pragma
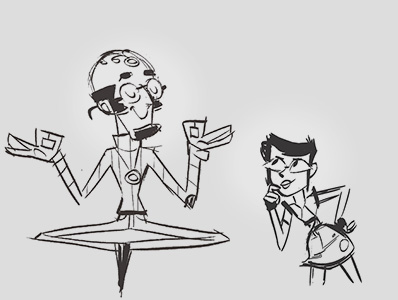
(SHOW YOUR TEACHER)
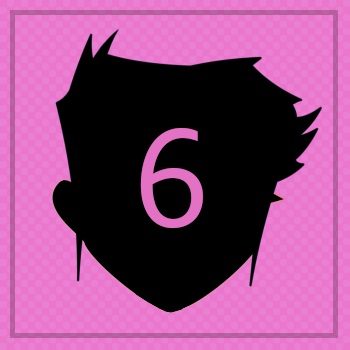